Overview
Concierge™ is a desktop hotel management application for receptionists to handle potential bookings and current guests. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Code contributed: [Code Contributed]
-
Major enhancements:
-
Implemented Autocomplete Feature
-
What it does: Ihe Autocomplete feature allows the user to seamlessly type in user commands with the autocompletion of an incomplete command that is input by the user. This feature helps the user by prompting the correct format.
-
Justification: This feature is not mandatory to have, however it would save the user a lot of time, especially if he/she is dealing with many different commands (in our case, many guests waiting to check in at the same time).
-
Highlights: It also eliminates the need for the user to remember all the prefixes because my implementation not only fills in the command, but fills in subsequent prefixes for the user as well which makes the feature more effective and efficient.
-
Credits: [Idea] Got the idea of implementing the autocomplete feature replete with prefixes and the various parameters pre-filled in from the link given. However, I decided to implement it with a Control button press so as to minimise the user having to navigate back and forth in the Command Box and it will also make for quicker typing once the user gets a flow of the autocomplete feature.
-
-
-
Minor enhancements:
-
Implemented Command Archive feature (Shifted to v2.0)
-
What it does: This feature keeps an archive of all the commands (both valid and invalid) input by a user and adds a time stamp plus user tag. This is different from the history command because this information will be exported into a txt/xml file instead of being destroyed at the end of the session as is seen in AB4
-
Justification: This feature is not mandatory, however it would serve an important purpose to hotel managers or the person-in-charge. This feature is aimed at tracking and identifying any suspicious activity and also to serve as data that could either be used in the future for monthly audits and even possibly generating statistics as to what items/facilities are patronised most by the guests.
-
-
-
Other contributions:
-
Setting up the initial user guide with all the new commands we aimed to implement
-
PRs reviewed (with some review comments): #133, #139, #140, #143, #153, #162, #191, #229, #235, #236
-
Reported bugs and suggestions for other teams in the class: unclear usage of find feature, unable to edit multiple parameters at once, unclear purpose of back command /bk prompt of add command
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Autocomplete: Ctrl, Alt
Auto-completes a partially typed in command by a user.
Function Press Alt to quick-clear the CommandBox
(saves time
for user when he wants to clear the box).
The command box before Alt is pressed:

The command box aft Alt is pressed:

Format: Enter COMMAND_WORD
, followed by Ctrl key.
Function: Press Ctrl key again to proceed to the next prefix.
Example:
Step 1: User enters a
in CommandBox
.

Step 2: After Ctrl has been pressed, it automatically inserts the
command and the first parameter PREFIX_NAME
in the command line.

Step 3: After filling up the name field, e.g. with Anthony, then press
Space. Finally, to insert the next parameter PREFIX_PHONE
press Ctrl.

Step 4: Repeat Step 3 until all the parameters are input, then press Enter to execute the command.

Note(s):
-
For finishing entering a prefix field e.g. 'n/John Doe', user has to insert Space on his/her own before pressing Ctrl again, to autocomplete the next prefix.
-
For commands such as
checkin
andcheckout
, user has to specify up till at leastchecki
orchecko
because the application is unable to distinguish from the two commands without sufficient information. The subsequent prefixes will follow suit accordingly.-
archive
- Opens the log where all user input is captured and saved. All keystrokes are captured, including invalid/mistyped and login commands.
-
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Autocomplete: Ctrl, Alt
Overview
The Autocomplete feature allows the user to seamlessly type in the full command and prefixes without having to worry if he/she missed out on any prefix. This feature helps the user by prompting the correct format. This is useful as some of the commands require several inputs from the user and hence this will save time and commands can be executed faster.
A quick-clear has also been added as part of this feature, so that
the user can again, save time.Press Alt to quick-clear the
CommandBox
(saves time for user when he wants to clear the box).
The command box before Alt is pressed:

The command box aft Alt is pressed:

Example of how feature works
Step 1: Launch application
Step 2: User enters a
in CommandBox
then presses Ctrl.
AutoCompleteManager()
compares input
through the list of
initCommandKeyWords`and proceeds to display the command in the
`CommandBox
because a
is an applicable COMMAND_WORD
.

After Ctrl has been pressed, it automatically inserts the
first prefix PREFIX_NAME
in the command line.

Step 3: After the user fills up the PREFIX_NAME
field, he can press
Space to move on to the next prefix. After pressing Space, then
he can press Ctrl. At this point, AutoCompleteManager()
is
called again but this time instead of calling the getAutoCompleteCommands()
it calls getAutoCompleteNextMissingParameter
since it will detect
the presence of the PREFIX_NAME
parameter.
This is the expected outcome before pressing Ctrl

This is the expected outcome after pressing Ctrl

Step 4: The user repeats Step 3 until all parameters are input by the user
and then presses Enter to execute the command.
Note: For AddCommand
, the final parameter PREFIX_TAG
is optional, so
the user can just delete it if he chooses not to add a tag.
This is the expected outcome after all the parameters are filled.

Press Enter to execute the command.
Given below is the activity diagram for the Autocomplete feature.
Activity Diagram :
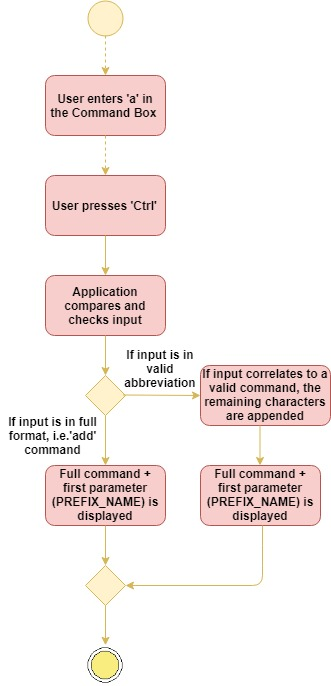
Activity Diagram demonstrates what happens when user presses Ctrl
Current Implementation
The Autocomplete mechanism is facilitated by AutoCompleteManager
, which
can be found in LogicManager
. It supports the auto completion of incomplete
commands by providing a list of auto completed commands from a given incomplete
command.
An underlying Trie
data structure is used to facilitate the AutocompleteManager
.
Trie
only supports autocompletion of commands that are provided by the
AutocompleteManager
. The CommandParameterSyntaxHandler
that is found in
AutocompleteManager
supports the autocompletion of parameters for commands.
Given below is the class diagram for the Autocomplete feature.
Autocomplete Class Diagram :
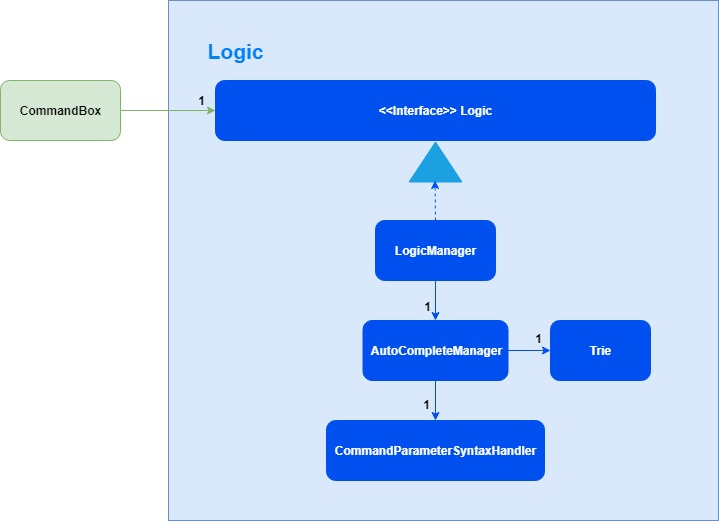
The CommandBox
interacts with the AutocompleteManager
using LogicManager
.
When the user presses Ctrl in the command box, the CommandBox
will
handle the Ctrl key press event and will execute the AutoCompleteUserInput()
method.
Given below is the sequence diagram for the Autocomplete feature.
Autocomplete Sequence Diagram :
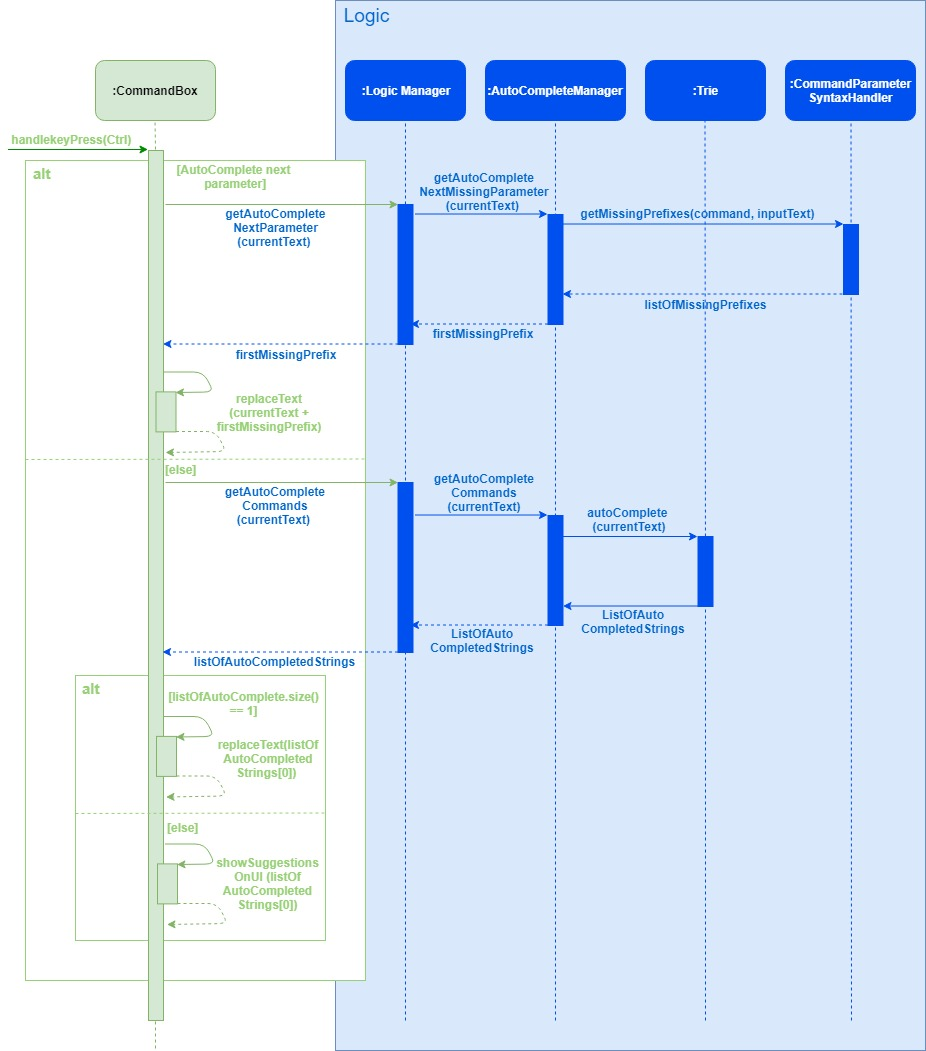
Design Considerations
Aspect: Implementation of Autocomplete
-
Alternative 1 (current choice): Use a manager (
AutoCompleteManager
) to handle the autocomplete helper methods.-
Pros: Allows for better usability and more code abstraction.
-
Cons: The amount of time taken for a new developer to to understand all the interaction between methods will be longer.
-
-
Alternative 2: Iterate through all possible commands to find match prefix.
-
Pros: Implementation of this alternative would be easier.
-
Cons: If there are too many commands being input consecutively, the application might start to lag due to possible loss of performance.
-
Aspect: Implementation of Algorithm
-
Alternative 1 (current choice): Trie Data Structure
-
Pros: Performance of application will be better.
-
Cons: The complexity of implementation is higher.
-
-
Alternative 2: Iterate through all possible commands to find match prefix.
-
Pros: Implementation of this alternative would be easier.
-
Cons: If there are too many commands being input consecutively, the application might start to lag due to possible loss of performance.
-
[Proposed] Command Archive feature
Given below is the UML diagram for the CommandArchive
Class:
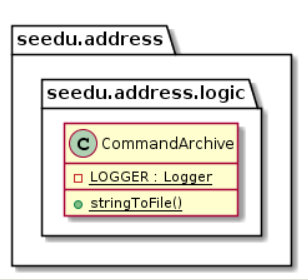
Given below is the UML diagram for the CommandHistory
Class:
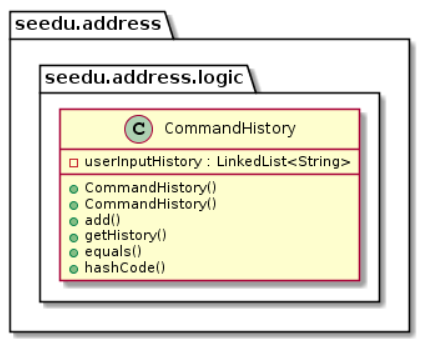
Current Implementation
The Command Archive mechanism is facilitated by CommandArchive
.
It utilises the userInputHistory
to extract the latest command that the user
has input and passes the inputString`to `stringToFile
method in CommandArchive
class. The inputString
is then appended to the CommandFile.txt
file.
Additionally, it implements the following operations:
-
StringBuilder()
— The main operations of theStringBuilder
are theappend
andinsert
methods which can be overloaded to accept data of any type. Theappend
method always adds these characters at the end of the builder.This operation can be found inCommandHistory
. -
toString()
— Converts the StringBuilder object into a string namedinputString
so it can be passed to theCommandArchive
class. This operation can be found inCommandHistory
.*getLogger()
— CreatesLOGGER
so that it can log anyIOExceptions
that are caught in the catch blocks of the methods found instringToFile
method ofCommandArchive
. -
substring()
— Extracts the latest command from theuserInputHistory
. This is required because theuserInputHistory
appends all the older commands into the LinkedList as well. This is done by looking for the first newLine character occurrence of theinputString
. The substring is then extracted aslatestUserCommand
. This operation can be found inCommandArchive
. -
simpleDateFormat()
— Creates atimeStamp
in DD/MM/YYY format that can later be appended tolatestUserCommand
. This operation can be found inCommandArchive
. -
fileWriter
— Writes the stream of characters (which islatestUserCommand
) tocommandHistory
file. This will eventually be the output that is written intocommandFile.txt
viaPrintWriter
. ThePrintWriter
also appendstimeStamp
to the latest entry (which is eventuallytimeStamp
+latestUserCommand
). This operation can be found in CommandArchive`.This command requires a login.
Design Considerations
Aspect: How to extract userInputHistory
-
Alternative 1 (current choice):
userInputHistory
is first put into astringBuilder
and then converted to string to then pass toCommandArchive
.-
Pros:
-
Easy to implement because
StringBuilder
can utiliseappend
andinsert
methods, which can be overloaded to accept any data. -
Faster than
StringBuffer
under most implementations. -
StringBuilder is mutable while String is immutable.
-
-
Cons: String is more optimised especially if you don’t need the extra features of
StringBuilder
-
-
Alternative 2: Create a KeyLogger class that implements KeyListener to capture userInput.
-
Pros: It is more secure and can only be accessed for audits and other administrative access purposes and is hidden from the user.
-
Cons:
-
If implemented wrongly, it will become a global KeyLogger that captures userInput outside of application.
-
Does not utilise the existing infrastructure and data found in the base level program class
CommandHistory
and hence would require more effort to implement.
-
-