Overview
Concierge is a desktop application simulating a hotel management system used by hotel owners and receptionists. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Code contributed: [Code Contributed]
-
Major enhancements:
-
Expanding on the existing
list
function:-
What it does: It allows the user to list between seeing a list of all guests, list of checked-in guests, or a list of all the rooms in the hotel.
-
Justification: This feature is essential to a proper, working hotel management system. The listing of information on guests and rooms is needed for receptionists to perform follow-up tasks, be it in person or within the app.
-
Highlights: The listing function was made with the intention of listing guests and rooms separately under the same column by modifying JAVAFX and FXML files.
-
Credits: Additional credit should also be given to my groupmate Adam Chew (adamwth), who added the functionality of list checked-in guests with the
list -cg
command.
-
-
Expanding on the existing
find
function:-
What it does: It allows the user to search for all guests, checked-in guests, or rooms based on a particular set of conditions/predicates related to the attributes of the guest/room.
-
Justification: This feature is essential to a proper, working hotel management system. The find function can be used in a multitude of scenarios, such as when a quick search of rooms of an exact capacity is needed, or when a checked-in guest needs to be located immediately, in the event of an emergency.
-
Highlights: The prefixes of the find function can be chained in any order, and in any quantity, as the predicates are all combined at the end. Each filter is has an "and" relationship with one another.
-
Credits: The modification of the find function was dependent on its own initial code, and the style of coding and arriving at the solution was closely followed.
-
-
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Locating guests and rooms : find
Finds guests or rooms, depending on the input flag and the keyword filters.
Format: find FLAG FILTER [MOREFILTERS]
Filters for Guest / Checked-in Guests (-g/-cg):
-
n/
- Name -
p/
- Phone Number -
e/
- Email Address -
t/
- Tags
Filters for Rooms (-r):
-
r/
- Room Number -
c/
- Capacity -
t/
- Room Tags -
n/
- Guest name within bookings -
-hb
- Has Bookings Flag* -
-nb
- No Bookings Flag* -
from/
- Booking Start Date^ -
to/
- Booking End Date^
Flags marked with * cannot be used together in the same command. |
Examples:
-
find -g n/Alex Yu
Find guest(s) with "Alex" or "Yu" or both in their names. -
find -g n/Alex t/VIP
Find guest(s) named Alex with tag "VIP". -
find -cg p/81027115
Find checked-in guest(s) with phone number "81027115". -
find -cg t/VIP
Find checked-in guest(s) with tag "VIP". -
find -r r/085
Find room 085 -
find -r c/2
Find all rooms with a capacity of 2. -
find -r c/5 -nb from/ 01/11/2018 to/ 05/11/2018
Find all rooms with a capacity of 5, without any bookings from the date range 01/11/2018 to 05/11/2018. -
find -r -hb
Find all rooms with bookings. -
find -r -hb t/RoomService
Find all rooms with bookings with tag "RoomService".
Listing all guests : list
Shows the entire list of rooms, checked-in guests, or archived guests, depending on the input flag
Format: list FLAG
Examples:
-
list -r
List all rooms -
list -g
List all archived guests -
list -cg
List all checked-in guests
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Find Feature
The Find feature expands upon the originally implemented FindCommand, allowing for the searching of both rooms and guests, with several filters.
The Activity UML Diagram for the current implementation of FindCommand is as follows:
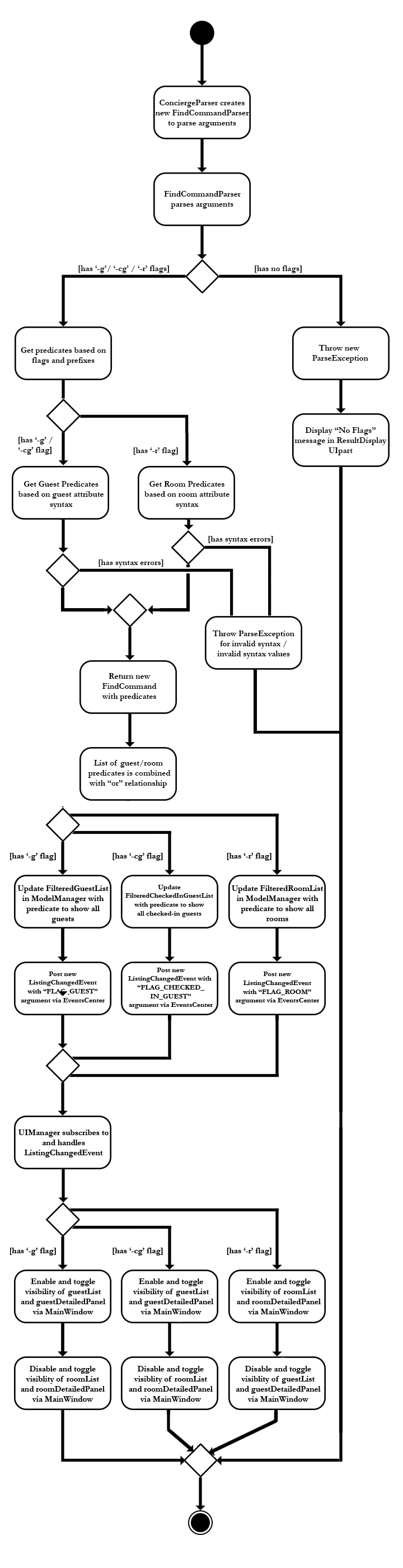
Current Implementation
The find function is facilitated by a modified FindCommand
class, of which the input from the CommandBox is parsed by a FindCommandParser
class.
The find function now has the ability to find either guests or rooms. The starting commands for the find function with flags are as follows:
-
find -g
- Find guests. -
find -cg
- Find checked-in guests. -
find -r
- Find rooms.
The above command must be followed up by at least 1 filter, and they are as follows:
Guest Filters (-g):
-
n/ - Name
-
p/ - Phone Number
-
e/ - Email Address
-
t/ - Tags
Room Filters (-r):
-
r/ - Room Number
-
c/ - Capacity
-
t/ - Room Tags
-
n/ - Name of guest with bookings
The following are filters for room bookings. The flags cannot be mixed. The flags can be used independently, or with a from/to specified date. Input dates must be in DD/MM/YY format.
-
-hb - Has Bookings Flag
-
-nb - No Bookings Flag
-
from/ - Booking Start Date
-
to/ - Booking End Date
The FindCommandParser uses a tokenizer to obtain the individual arguments/filters, whether the filter is present or not. If a filter is present, the input that precedes the filter prefix will be used to create the individual predicate class.
These predicate classes are collected into a list of predicates before they are combined and merged in the FindCommand class. The combined final predicate is then passed to the Model Manager to filter the guest/room list, and a listingChangedEvent is called to update the UI elements.
Design Considerations
Aspect: OR/AND Searching
When searching, a few things have to be considered. Does the filter specified have an OR relationship with one another, or an AND relationship. An example is this: find -g n/Alex t/VIP, this can be interpreted in two ways. Finding guests with name as "Alex" AND with tag "VIP", or name "Alex" or tag "VIP.
Rooms List Feature
The rooms list feature builds upon, and reuses functions from the ;originally implemented ListCommand.
The Activity UML Diagram for the current implementation of ListCommand is as follows:
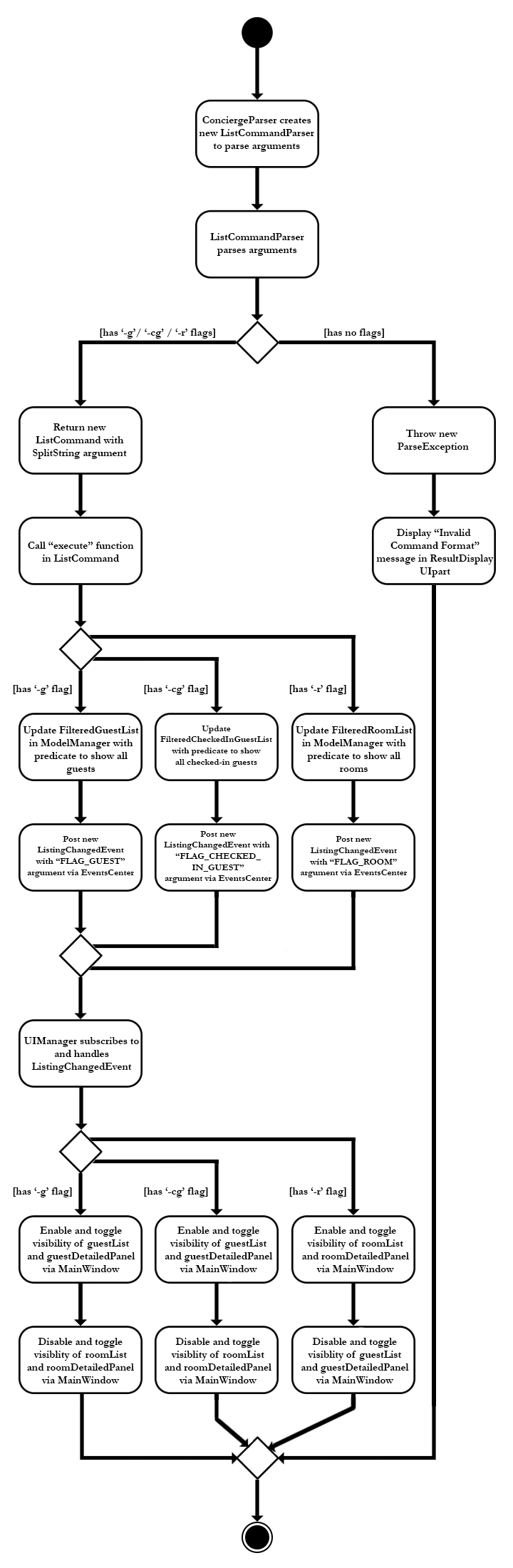
Current Implementation
The list function is facilitated by a modified ListCommand
class, of which the input from the CommandBox is parsed by a ListCommandParser
class.
The list function now requires a flag after the 'list' command. Below are the two allowed list commands:
-
list -g
- Lists all guests. -
list -cg
- Lists all checked-in guests. -
list -r
- Lists all rooms.
A ListCommandParser
class was created to obtain and compare the flags from inputs, which required a different approach to the rest of the commands. The input string is simply split using a String function, obtaining an array of strings, of which the flags will be at index 1.
Modification of existing FXML files, and creation of new FXML files was done to achieve separate listing of guests and rooms, and the browser panel was replaced with a panel to focus on, and display more detailed information on the selected guest/room.
In order to stack the UI elements on top of one another to reuse and display the separate lists under the same column, modifications were made to the MainWindow.fxml
file.
The GuestListPanel and RoomListPanel each has a "VBox" element encapsulating them, which visibility is toggled and the element itself enabled or disabled based on the flag that was obtained from the parser. This feature extends to the GuestDetailedPanel and RoomDetailedPanel and is achieved in the same way.
Design Considerations
Aspect: How to display each list
-
Alternative 1 : Maintain two columns on the MainWindow UI to display both rooms and guests
-
Pros: Easier to modify UI by adding on instead of modifying and replacing, and modifications in the future will not be too tedious.
-
Cons: UI looks cluttered with an empty column when not displaying the other, not an efficient use of screen space.
-
-
Alternative 2 (current choice): Separately display the two lists within the same MainWindow UI space/column.
-
Pros: Cleaner looking, fully utilises empty spaces. Better visual feedback from commands as inputs.
-
Cons: Requires heavy modification of MainWindow UI files, future features must stick with the restriction of having a list of either guests or rooms.
-